Apitally now supports AdonisJS
Introducing our new integration for AdonisJS, offering comprehensive API insights and request logging with minimal effort.

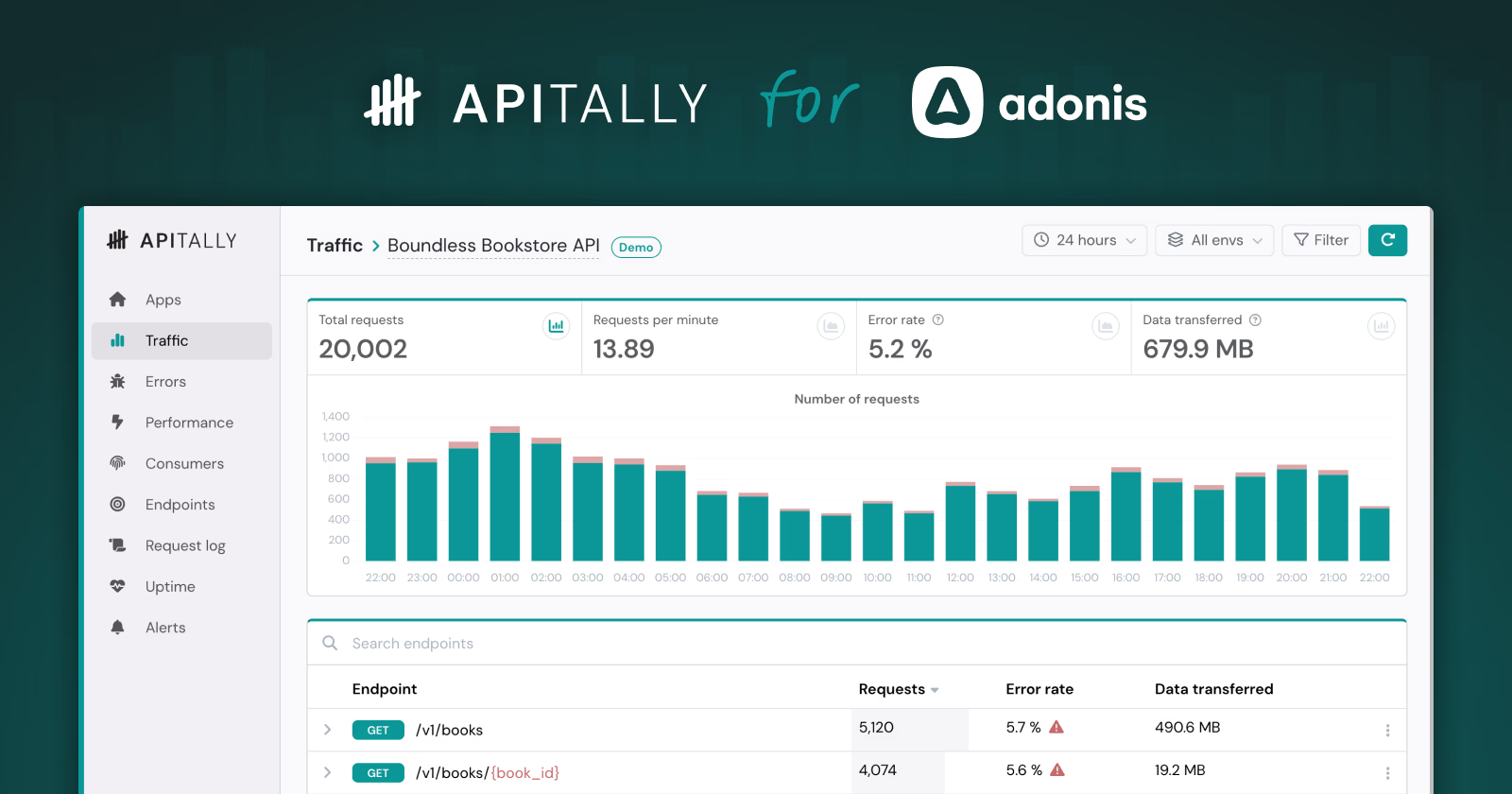
We’re excited to announce that Apitally now supports AdonisJS.
AdonisJS is a full-featured, TypeScript-first Node.js framework designed for building scalable and maintainable web applications and APIs. What sets it apart from other frameworks is its deep focus on developer productivity, offering a convention-over-configuration approach similar to Laravel. It even has its own CLI tool and VSCode extension!
Apitally in a nutshell
Aligning with AdonisJS’s focus on developer productivity, Apitally brings powerful API monitoring, analytics and request logging to your projects with just a few lines of code:
- Understand API usage and adoption
- Track client and server errors
- Monitor API performance
- Log, find and inspect individual API requests and responses
- Get notified when something goes wrong using synthetic health checks, heartbeats and custom alerts
Apitally provides intuitive dashboards for your APIs out of the box, so you don’t have to spend time building your own. The dashboards give you a clear overview of what’s happening with your API, while allowing you to drill down into any area of interest.
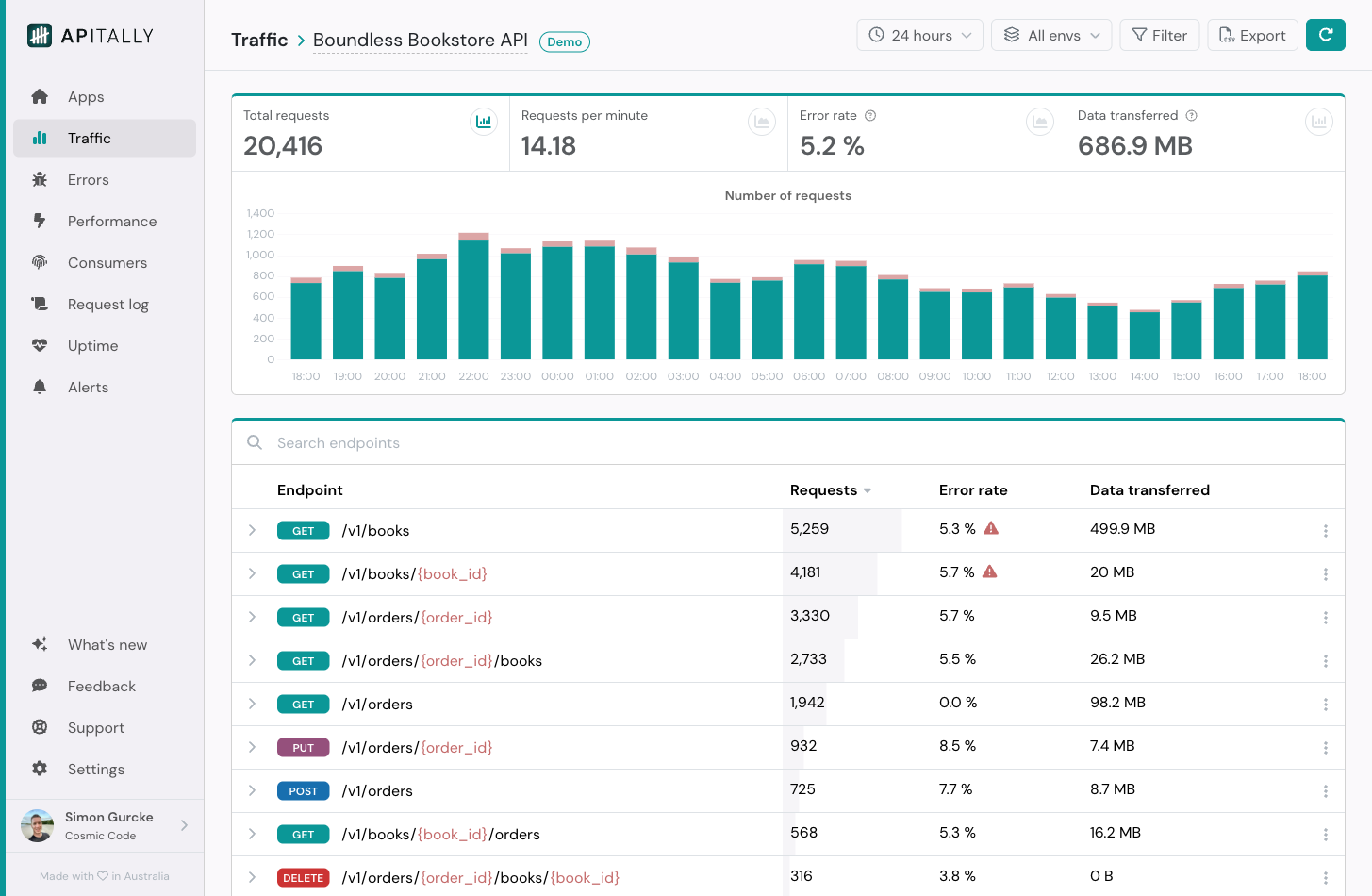
How it works
Apitally’s open-source SDK integrates with AdonisJS using a lightweight middleware which automatically captures key API metrics for each request and response. The middleware times the invocation of your route handlers and collects metadata about requests and responses, including method, path, status code, and validation errors.
The SDK aggregates this data client-side and sends it to the Apitally dashboard in the background at regular intervals, ensuring minimal impact on your application’s performance.
By default, Apitally doesn’t capture any sensitive information, making it suitable for applications with strict privacy requirements. If you need more detailed insights, request logging is available as an opt-in feature that you can configure to match your specific requirements.
Getting started
Setting up Apitally for your AdonisJS project only takes a few minutes.
-
The first step is to grab a client ID by signing up and creating an app in the Apitally dashboard.
-
Then, add the
apitally
package to your project’s dependencies:
npm install apitally
- Create a configuration file at
config/apitally.ts
with your client ID:
import { defineConfig } from 'apitally/adonisjs'
const apitallyConfig = defineConfig({
clientId: 'your-client-id', // provided in Apitally dashboard
env: 'prod', // or 'dev' etc.
})
export default apitallyConfig
- Register the Apitally provider in your
adonisrc.ts
file:
import { defineConfig } from '@adonisjs/core/app'
export default defineConfig({
// ... existing code ...
providers: [
// ... existing providers ...
() => import('apitally/adonisjs/provider'),
],
})
- Add the Apitally middleware in your
start/kernel.ts
file:
import router from '@adonisjs/core/services/router'
router.use([
() => import('apitally/adonisjs/middleware'),
// ... other middleware ...
])
- Finally, to capture validation and server errors, modify your exception handler to call the
captureError
function:
import { ExceptionHandler, HttpContext } from '@adonisjs/core/http'
import { captureError } from 'apitally/adonisjs'
export default class HttpExceptionHandler extends ExceptionHandler {
// ... existing code ...
async report(error: unknown, ctx: HttpContext) {
captureError(error, ctx)
return super.report(error, ctx)
}
}
After deploying your application, you should start seeing data in the Apitally dashboard within a few seconds.
Identifying consumers
Apitally allows you to track API usage per consumer. To enable this, you can identify consumers in your application by setting the apitallyConsumer
property on the ctx
object.
If your application uses authentication, it makes sense to set this property based on the authenticated user. You could do this in a custom middleware, for example.
import { HttpContext } from '@adonisjs/core/http'
import { NextFn } from '@adonisjs/core/types/http'
export default class ApitallyConsumerMiddleware {
async handle(ctx: HttpContext, next: NextFn) {
if (ctx.auth.isAuthenticated) {
ctx.apitallyConsumer = ctx.auth.user!.email
}
await next()
}
}
Conclusion
With Apitally’s new AdonisJS integration, you can gain valuable insights into your API’s usage, errors and performance with minimal effort. This allows you to stay productive and focus on building great APIs rather than setting up complex monitoring infrastructure.
For further instructions, including how to configure request logging, check out our comprehensive setup guide for AdonisJS.
Happy monitoring!